thermography.classification.models package¶
The models (computation graphs) which can be used to train a classifier are coded here.
Submodules¶
thermography.classification.models.base_net module¶
-
class
BaseNet
(x: tensorflow.python.framework.ops.Tensor, image_shape: numpy.ndarray, num_classes: int, name: str = 'ThermoNet')[source]¶ Bases:
abc.ABC
Base interface for nets used by the
thermography
package. This class offers a convenience interface to train a classifier and to access its data.-
channels
¶ Returns the number of channels accepted by the model.
-
create
() → None[source]¶ Method which each subclass must implement which creates the computational graph.
Note
This method must use the input placeholder
self.x
and terminate into the logits tensorself.logits
.
-
flat_shape
¶ Returns the shape of the image which the model takes as input to the computational graph.
Example: img_batch.shape = [batch_shape, w, h, c] flat = model.flat_shape # [w, h]
-
image_shape
¶ Returns the image shape accepted by the model. This shape corresponds to the shape of each element carried by the input placeholder
self.x
.
-
logits
¶ Returns a tf.Tensor representing the logits of the classified input images.
-
name
¶ Returns the name associated to the model.
-
num_classes
¶ Returns the number of classes which the model will consider for classification.
-
static
update_shape
(current_shape: numpy.ndarray, scale: int) → numpy.ndarray[source]¶ Updates the current shape of the data in the computational graph when a pooling operation is applied to it.
Example: current_shape = self.flat_shape # [w, h] conv = conv_relu(x=self.x, kernel_shape, bias_shape) pool = max_pool_2x2(name="max_pool", x=conv) current_shape = self.update_shape(current_shape, 2) # [w/2, h/2]
-
x
¶ Returns a reference to the input placeholder.
-
thermography.classification.models.thermo_net module¶
thermography.classification.models.thermo_net_3x3 module¶
-
class
ThermoNet3x3
(x: tensorflow.python.framework.ops.Tensor, image_shape: numpy.ndarray, num_classes: int, keep_prob: float, *args, **kwargs)[source]¶ Bases:
thermography.classification.models.base_net.BaseNet
Class representing the computational graph structure used for classification of solar panel modules.
Initializes the computational graph according to the structure defined in
self.create
.Parameters: - x – Tensorflow placeholder which will be fed with a batch of images.
- image_shape – Shape of each input image fed to
self.x
. - num_classes – Number of classes which the model can predict.
- keep_prob – Tensorflow placeholder representing the keep probability for the dropout layers.
Thermonet3x3 Structure¶
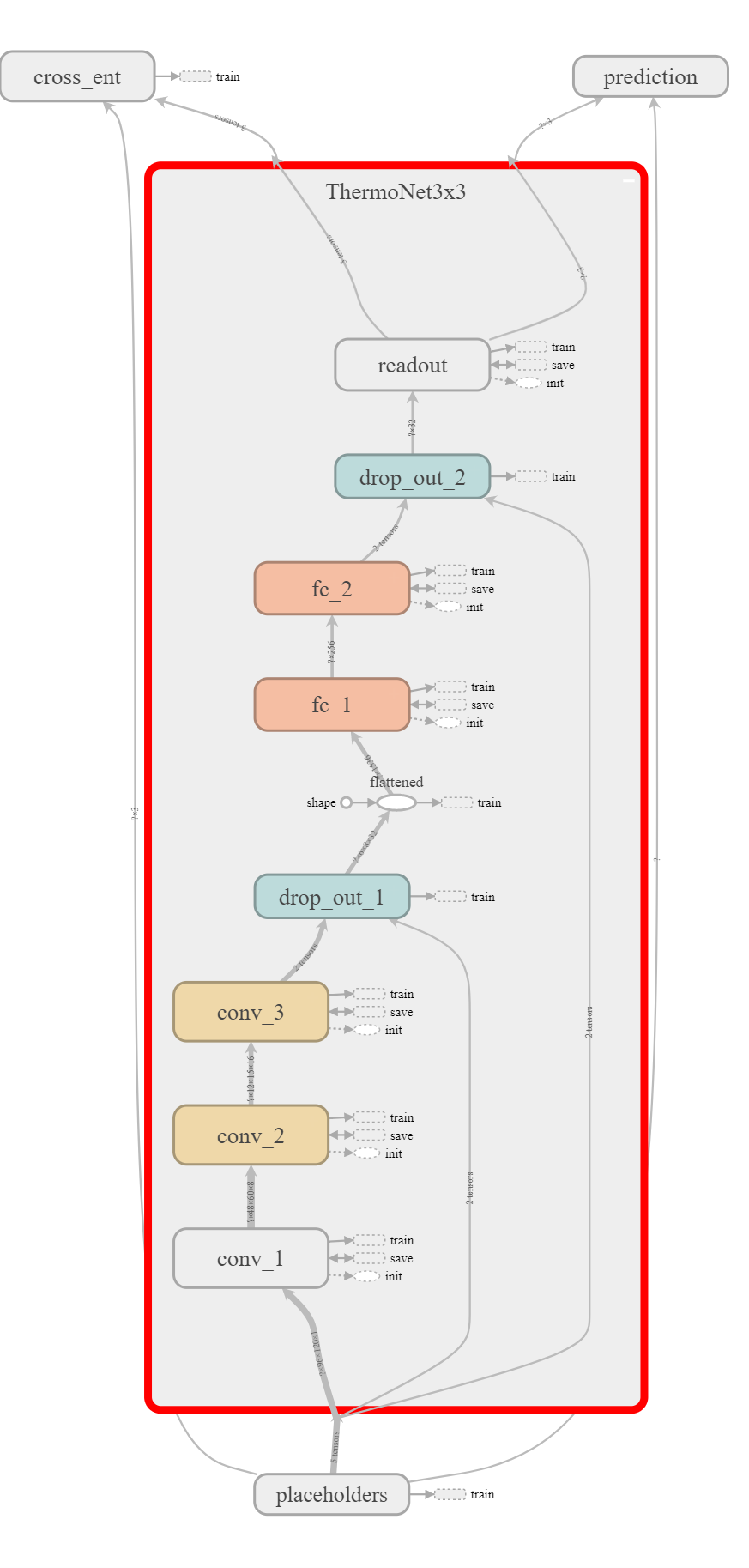